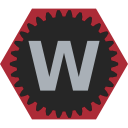 |
WPILibC++ 2025.2.1
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
7#ifndef WPI_MEMORY_CONFIG_HPP_INCLUDED
8#define WPI_MEMORY_CONFIG_HPP_INCLUDED
13#define WPI_MEMORY_IMPL_IN_CONFIG_HPP
15#undef WPI_MEMORY_IMPL_IN_CONFIG_HPP
19#ifndef WPI_HAS_EXCEPTION_SUPPORT
20#if defined(__GNUC__) && !defined(__EXCEPTIONS)
21#define WPI_HAS_EXCEPTION_SUPPORT 0
22#elif defined(_MSC_VER) && !_HAS_EXCEPTIONS
23#define WPI_HAS_EXCEPTION_SUPPORT 0
25#define WPI_HAS_EXCEPTION_SUPPORT 1
29#if WPI_HAS_EXCEPTION_SUPPORT
30#define WPI_THROW(Ex) throw(Ex)
33#define WPI_THROW(Ex) ((Ex), std::abort())
37#ifndef WPI_HOSTED_IMPLEMENTATION
38#if !_MSC_VER && !__STDC_HOSTED__
39#define WPI_HOSTED_IMPLEMENTATION 0
41#define WPI_HOSTED_IMPLEMENTATION 1
46#define WPI_MEMORY_LOG_PREFIX "wpi::memory"
49#define WPI_MEMORY_VERSION \
50 (WPI_MEMORY_VERSION_MAJOR * 100 + WPI_MEMORY_VERSION_MINOR)
55#ifndef WPI_IMPL_DEFINED
56#define WPI_IMPL_DEFINED(...) __VA_ARGS__
63#define WPI_EBO(...) __VA_ARGS__
66#ifndef WPI_ALIAS_TEMPLATE
73#define WPI_ALIAS_TEMPLATE(Name, ...) \
74 class Name : public __VA_ARGS__ \
78#define WPI_ALIAS_TEMPLATE(Name, ...) using Name = __VA_ARGS__
87#define WPI_MEMORY_VERSION_MAJOR 1
91#define WPI_MEMORY_VERSION_MINOR 1
95#define WPI_MEMORY_VERSION \
96 (WPI_MEMORY_VERSION_MAJOR * 100 + WPI_MEMORY_VERSION_MINOR)
101#define WPI_MEMORY_CHECK_ALLOCATION_SIZE 1
105#define WPI_MEMORY_DEBUG_ASSERT 1
109#define WPI_MEMORY_DEBUG_FILL 1
114#define WPI_MEMORY_DEBUG_FENCE 1
118#define WPI_MEMORY_DEBUG_LEAK_CHECK 1
122#define WPI_MEMORY_DEBUG_POINTER_CHECK 1
127#define WPI_MEMORY_DEBUG_DOUBLE_DEALLOC_CHECK 1
134#define WPI_MEMORY_NAMESPACE_PREFIX 1
144#define WPI_MEMORY_TEMPORARY_STACK_MODE 2